SaltForTheThirsty
AnetA8Drill()
Converted my Anet A8 to a CNC so I can drill PCBs. Hardware conversion was simple. I removed the hotend, associated fans, and hardware. I then drilled a hole big enough for the end of a RS-540 motor to go into and two holes for the motor mounting screws. I put a chuck on the end of the motor and I powered it up with a bench top power supply. I'm using Klipper firmware in my A8. This firmware doesn't work with the gcode generated by pcb2gcode so I wrote some software to convert it to gcode the firmware can work with.
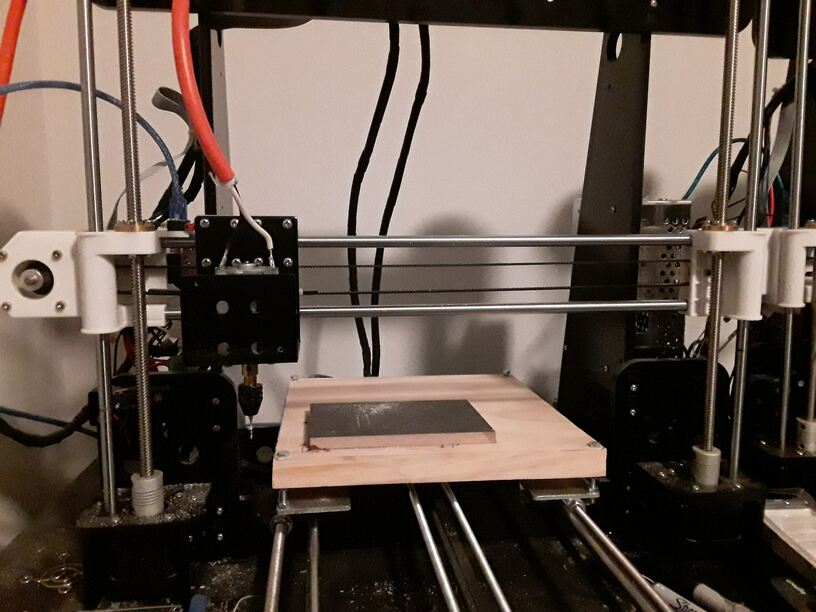
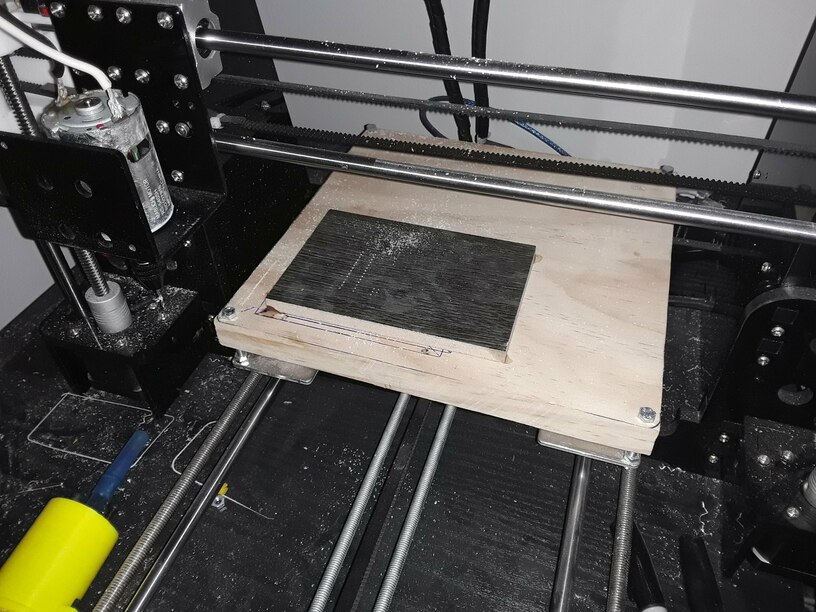
AnetA8Drill:
Download Files.#include <stdio.h> void downUp(float, float, float, float); int main(int argc, char **argv){ const float PCB_BLANK_X = 101.6; // size of pcb const float PCB_BLANK_Y = 76.2; // size of pcb const float BOARD_SIZE_X = 63.0; // size of board outline const float BOARD_SIZE_Y = 61.0; // size of board outline const float INITIAL_XOFFSET = 4.0; // how far the board is from the drills x = 0 coordinate const float INITIAL_YOFFSET = 24.0; // how far the board is from the drills y = 0 coordinate const float XYSPEED = 4800.0; const float ZSPEED = 100.0; const float ZDOWN = -5.0 ; const float ZUP = 5.0; const float INITIAL_ZOFFSET = 11.0; const float BITLENGTHBELOW = 15.0; const float ZSAFETYLENGTH = 2.0; const float FINAL_Z_OFFSET = INITIAL_ZOFFSET + BITLENGTHBELOW + ZSAFETYLENGTH; float finalXoffset, finalYoffset, centerX, centerY; centerX = (PCB_BLANK_X/2.0) + INITIAL_XOFFSET ; centerY = (PCB_BLANK_Y/2.0) + INITIAL_YOFFSET; finalXoffset = centerX - (BOARD_SIZE_X/2.0); finalYoffset = centerY - (BOARD_SIZE_Y/2.0); int c, d; d = 'a'; // initialize to something other than '\n' or EOF // preamble printf("; centerX = %f\tcenterY = %f\n", centerX, centerY); printf("; finalXoffset = %f,\tfinalYoffset = %f\n", finalXoffset, finalYoffset); printf("G21 ;metric values\n" "G90 ;absolute positioning\n" "M107 ;start with the fan off\n" "G28 X0 Y0 ;move X/Y to min endstops\n" "G28 Z0 ;move Z to min endstops\n" "G1 Z15.0 F9000 ;move the platform down 15mm\n" "G1 F9000\n" // Put printing message on LCD screen "M117Drilling...\n" "G92 E0\n" "G1 F%f\n" "M107\n" "G1 Z%f\n" "G1 X%f Y%f\n" "G1 Z%f\n" "G92 X0 Y0 Z0\n" // "M206 X%f\n" // "M206 Y%f\n" /*"M206 Z%f\n" */, XYSPEED,FINAL_Z_OFFSET*2.0, finalXoffset, finalYoffset, FINAL_Z_OFFSET); printf("\n\n"); printf("G1 "); c = getchar(); while(!(d == '\n' && c == EOF)){ if(c != '\n'){ putchar(c); } else{ putchar(c); downUp(ZDOWN, ZUP, ZSPEED, XYSPEED); } d = c; c = getchar(); } printf("G28 X0 Y0 Z0\n"); return 0; } void downUp(float downPosition, float upPosition, float zSpeed, float xySpeed){ printf("G1 Z%f F%f\n",downPosition, zSpeed); printf("G1 Z%f F%f\n",upPosition, xySpeed); printf("G1 F%f", xySpeed); }